This function generates a Uniform sampling between
u = uniform_sampling(parameters, method, n_samples, seed)
Input variables
Name | Description | Type |
---|---|---|
parameters | Dictionary of parameters for uniform distribution. Keys:
| Dictionary |
method | Sampling method. Supports the following values:
| String |
n_samples | Number of samples | Integer |
seed | Seed for random number generation. Use None for a random seed | Integer or None |
Output variables
Name | Description | Type |
---|---|---|
u | Random samples | List |
Example 1
In this example, we will use the uniform_sampling
function from the parepy_toolbox
to generate two random samples (
# Libraries
import matplotlib.pyplot as plt
from parepy_toolbox import uniform_sampling
# Sampling
n = 400
x = uniform_sampling({'min': 10, 'max': 20}, 'mcs', n)
y = uniform_sampling({'min': 10, 'max': 20}, 'lhs', n)
# Plot
fig, axes = plt.subplots(1, 2, figsize=(7, 3))
sns.histplot(x, kde=True, bins=30, color='blue', ax=axes[0], alpha=0.6, edgecolor='black')
axes[0].set_title('MCS Sampling')
axes[0].set_xlabel('Values')
axes[0].set_ylabel('Density')
sns.histplot(y, kde=True, bins=30, color='green', ax=axes[1], alpha=0.6, edgecolor='black')
axes[1].set_title('LHS Sampling')
axes[1].set_xlabel('Values')
axes[1].set_ylabel('Density')
plt.tight_layout()
plt.show()
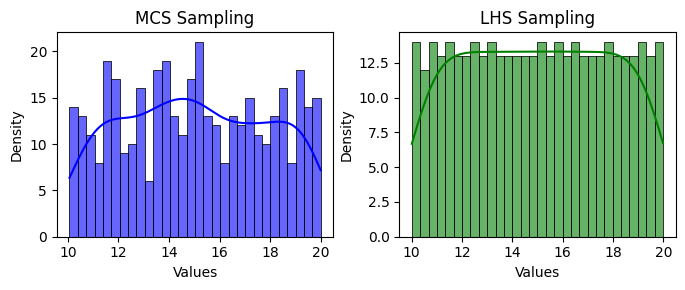
Figure 1. Uniform variable example.
Example 2
In this example, we will use the uniform_sampling
function from the parepy_toolbox
to generate two random samples (seed=25
), we generated 3 times and compared the results.
# Library
from parepy_toolbox import uniform_sampling
# Sampling
n = 3
x0 = uniform_sampling({'min': 10, 'max': 20}, 'mcs', n, 25)
x1 = uniform_sampling({'min': 10, 'max': 20}, 'mcs', n, 25)
x2 = uniform_sampling({'min': 10, 'max': 20}, 'mcs', n, 25)
print(x0, '\n', x1, '\n', x2)
Output details.
[11.607212332320078, 15.003120351710036, 12.16598464462817]
[11.607212332320078, 15.003120351710036, 12.16598464462817]
[11.607212332320078, 15.003120351710036, 12.16598464462817]
Note that using the seed 25 by 3 times, we can generate the same values in a random variable.