This function generates a Normal distribution with a specified mean \(\mu\) and standard deviation \(\sigma\).
u = normal_sampling(parameters, method, n_samples, seed)
Input variables
Name | Description | Type |
---|---|---|
parameters | Dictionary of parameters for the normal distribution. Keys:
| Dictionary |
method | Sampling method. Supports the following values:
| String |
n_samples | Number of samples | Integer |
seed | Seed for random number generation. Use None for a random seed | Integer or None |
Output variables
Name | Description | Type |
---|---|---|
u | Random samples | List |
Example 1
In this example, we will use the normal_sampling
function from the parepy_toolbox
to generate two random samples (\(n=400\)) following a normal distribution. The first set is sampled using the Monte Carlo Sampling (MCS) method, and the second using the Latin Hypercube Sampling (LHS) method. The mean and standard deviation are defined asĀ \([10, 2]\). The results are visualized using histograms with Kernel Density Estimates (KDE) plotted (using matplotlib lib) side-by-side for comparison.
# Libraries
import matplotlib.pyplot as plt
from parepy_toolbox import normal_sampling
# Sampling
n = 400
x = normal_sampling({'mean': 10, 'sigma': 2}, 'mcs', n)
y = normal_sampling({'mean': 10, 'sigma': 2}, 'lhs', n)
# Plot
fig, axes = plt.subplots(1, 2, figsize=(7, 3))
sns.histplot(x, kde=True, bins=30, color='blue', ax=axes[0], alpha=0.6, edgecolor='black')
axes[0].set_title('MCS Sampling')
axes[0].set_xlabel('Values')
axes[0].set_ylabel('Density')
sns.histplot(y, kde=True, bins=30, color='green', ax=axes[1], alpha=0.6, edgecolor='black')
axes[1].set_title('LHS Sampling')
axes[1].set_xlabel('Values')
axes[1].set_ylabel('Density')
plt.tight_layout()
plt.show()
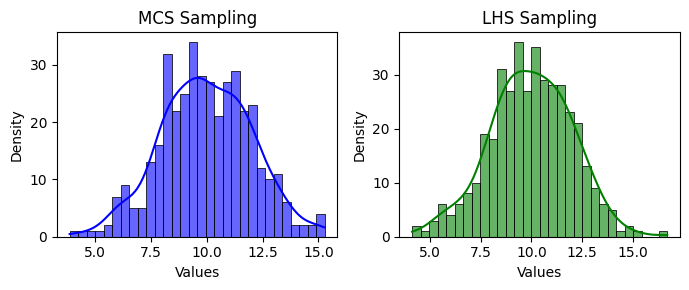
Figure 1. Normal variable example.
Example 2
In this example, we will use the normal_sampling
function from the parepy_toolbox
to generate two random samples (\(n=3\)) following a normal distribution. Using the Monte Carlo algorithm and the specific seed (seed=25
), we generated 3 times and compared the results.
# Library
from parepy_toolbox import normal_sampling
# Sampling
n = 3
x0 = normal_sampling({'mean': 10, 'sigma': 2}, 'mcs', n, 25)
x1 = normal_sampling({'mean': 10, 'sigma': 2}, 'mcs', n, 25)
x2 = normal_sampling({'mean': 10, 'sigma': 2}, 'mcs', n, 25)
print(x0, '\n', x1, '\n', x2)
Output details.
[11.607212332320078, 10.003120351710036, 12.16598464462817]
[11.607212332320078, 10.003120351710036, 12.16598464462817]
[11.607212332320078, 10.003120351710036, 12.16598464462817]
Note that using the seed 25 by 3 times, we can generate the same values in a random variable.